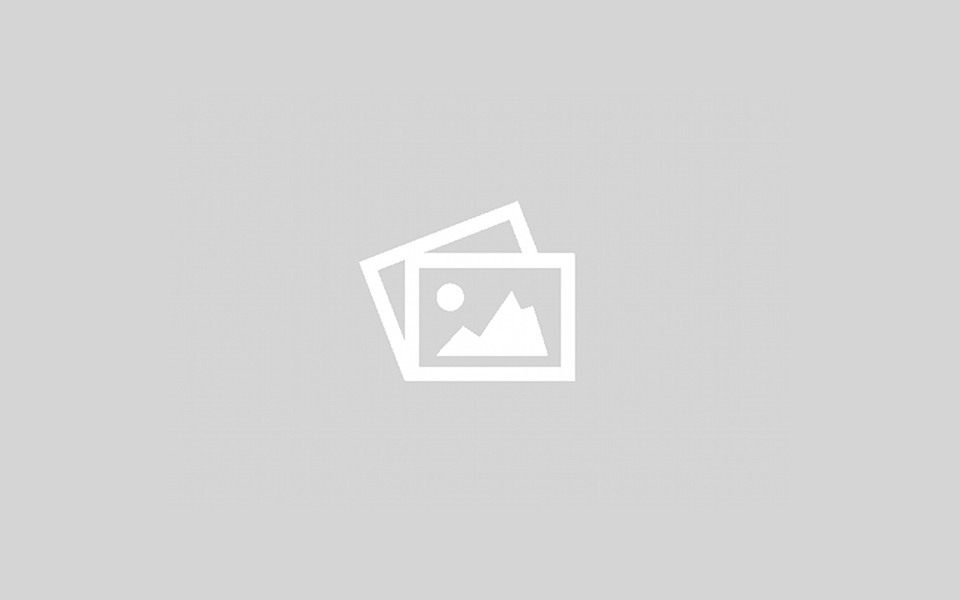
I was working on an autocomplete solution and I was thinking of ways to speed up the search. I will be providing a few examples with results below.
Q1 is the default wordpress search with no parameters. Q2 removes the caches since they wont be really necessary. And Q3 is tailored for precision; ie: my posts used [shortcode] to render almost the whole content dynamically, so I had almost no use for the_content . And you know WordPress by default, will search for both titles and the content. I thought why even bother.
Q1 resulted usually between 0.096 – 0.084, where Q2 took 0.064 – 0.061 . You see; you can shave almost 1/3 off by simply removing caches. As for Q3, I used $wpdb->get_result instead of WP_Query and typed in my exact sql in order to just search in titles and not the content. This reduced it even further and almost cutting the time in half, it was between 0.054 – 0.051.
You can get exactly same result in half the time.
Well, almost the same. Please note that Q3 will return everything as string, regardless of their type, that’s the only difference.
Bottomline is; WP_Query is very flexible, and usually effective, but it can always be a little more optimized depending on your needs just like in ‘if you need an id, just get an id’
Here are the queries for reference:
Q1 – Unoptimized Query
$req = 's'; $args = array( 'post_status' => 'publish', 'posts_per_page' => 20, 'numberposts' => 20, 's' =>$req, ); $acs_qy = new WP_Query($args); $acs_qy_arr = $acs_qy->posts;
Q2 – Optimized Query
$req = 's'; $args = array( 'post_status' => 'publish', 'posts_per_page' => 20, 'numberposts' => 20, 'cache_results'=>false, 'update_post_term_cache' => false, 'update_post_meta_cache' => false, 'no_found_rows' => true, 's' =>$req, ); $acs_qy = new WP_Query($args); $acs_qy_arr = $acs_qy->posts;
Q3 – Manual Query
$req = 's'; $mq = "SELECT wp_posts.* FROM wp_posts WHERE 1=1 AND wp_posts.post_title LIKE '%%$req%%' AND (wp_posts.post_status = 'publish') ORDER BY wp_posts.post_title LIKE '%%$req%%' DESC, wp_posts.post_date DESC LIMIT 0, 20"; $acs_qy_arr = $wpdb->get_results( $mq );