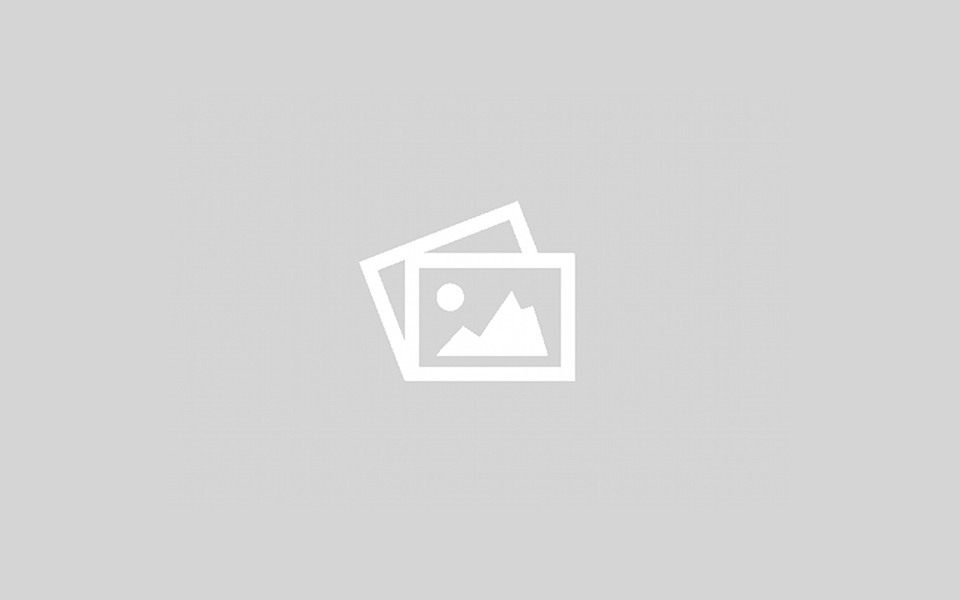
If you want to add name email or any additional fields in addition to password for protected posts, see the steps below:
/****************** custom protected post form *****/ add_filter( 'the_password_form', 'custom_password_form' ); function custom_password_form() { global $post; $label = 'pwbox-'.( empty( $post->ID ) ? rand() : $post->ID ); $a = '<div class="clearfix"><form id="password_form" class="protected-post-form" action="' . get_option('siteurl') . '/wp-login.php?action=postpass" method="post"> <label for="phone_input">Name: </label><input type="text" name="phone_input" /><br /> <label for="mail_input">Email: </label><input type="email" name="mail_input" /> ' . '<p>' . __( "Enter Password to view post:" ,'text-domain') . '</p>' . ' <label for="' . $label . '">' . __( "Password:" ,'text-domain') . ' </label><div class="input-append"><input name="post_password" id="' . $label . '" type="password" size="20" /><input type="submit" name="Submit" class="btn btn-primary" value="' . esc_attr__( "Gönder",'text-domain' ) . '" /></div> <input type="hidden" name="res_postid" value="' . $post->ID . '"> </form></div> '; return $a; }
Now to the validation
/** * Set the post password cookie expire time based on the email */ add_filter( 'post_password_expires', function( $valid ) { $postid = filter_input( INPUT_POST, 'res_postid', FILTER_SANITIZE_NUMBER_INT ); $email = filter_input( INPUT_POST, 'mail_input', FILTER_SANITIZE_STRING ); $name = filter_input( INPUT_POST, 'name_input', FILTER_SANITIZE_STRING ); $subpw = filter_input( INPUT_POST, 'post_password', FILTER_SANITIZE_STRING ); // a timestamp in the past $expired = time() - 10 * DAY_IN_SECONDS; if ( empty( $postid ) || ! is_numeric( $postid ) ) { // empty or bad post id, return past timestamp return $expired; } if ( empty($email) || ! filter_var($email, FILTER_VALIDATE_EMAIL) ) { // empty or bad email id, return past timestamp return $expired; } $the_post = get_post( $postid ); $postpw = $the_post->post_password; if($postpw !== $subpw) return $expired; //see below // pw_post_email_notify($name,$email); return $valid; }, 0 );
What you want to do with the information (name, email) is up to you. I wanted to be notified who logged in to view the post so I wrote a email notification as follows:
function pw_post_email_notify($name= NULL,$email= NULL) { $to = get_bloginfo('admin_email'); $blogname = get_bloginfo('blogname'); $frommail = $to; if ( ! isset($email) ) return false; $headers = sprintf( "From: %s <%s>\r\n", $blogname, $frommail ); $subject = "Someone has viewed the protected post"; $msg = "Name: " .$name. " - Email: ".$email; $mail_result = wp_mail( $to, $subject, $msg, $headers ); }